-
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
3ede1dc
commit 3675bff
Showing
7 changed files
with
577 additions
and
9 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,8 +1,246 @@ | ||
# notification | ||
# Notification | ||
|
||
## 安装 | ||
|
||
```shell | ||
composer require friendsofhyperf/notification | ||
composer require friendsofhyperf/notification:~3.1.0 | ||
``` | ||
|
||
## 使用 | ||
|
||
### 在 Model 中使用 `Notifiable` trait | ||
|
||
```php | ||
<?php | ||
|
||
declare(strict_types=1); | ||
|
||
namespace App\Model; | ||
|
||
use Hyperf\DbConnection\Model\Model; | ||
use FriendsOfHyperf\Notification\Traits\Notifiable;use Overtrue\EasySms\PhoneNumber; | ||
|
||
/** | ||
* @property int $id | ||
* @property \Carbon\Carbon $created_at | ||
* @property \Carbon\Carbon $updated_at | ||
*/ | ||
class User extends Model | ||
{ | ||
use Notifiable; | ||
|
||
/** | ||
* The table associated with the model. | ||
*/ | ||
protected ?string $table = 'user'; | ||
|
||
/** | ||
* The attributes that are mass assignable. | ||
*/ | ||
protected array $fillable = ['id', 'created_at', 'updated_at']; | ||
|
||
/** | ||
* The attributes that should be cast to native types. | ||
*/ | ||
protected array $casts = ['id' => 'integer', 'created_at' => 'datetime', 'updated_at' => 'datetime']; | ||
|
||
// 通知手机号 | ||
public function routeNotificationForSms(): string|PhoneNumber | ||
{ | ||
return $this->phone; | ||
} | ||
} | ||
``` | ||
|
||
### Database Notifications | ||
|
||
```shell | ||
# Install the database package | ||
composer require hyperf/database:~3.1.0 | ||
|
||
# Publish the migration file | ||
php bin/hyperf.php notification:table | ||
|
||
# Run the migration | ||
php bin/hyperf.php migrate | ||
|
||
# Create a notification | ||
php bin/hyperf.php make:notification TestNotification | ||
``` | ||
|
||
--- | ||
|
||
```php | ||
<?php | ||
|
||
namespace App\Notification; | ||
|
||
use FriendsOfHyperf\Notification\Notification; | ||
|
||
class TestNotification extends Notification | ||
{ | ||
public function __construct( | ||
private string $message | ||
){} | ||
|
||
public function via() | ||
{ | ||
return [ | ||
// database channel | ||
'database' | ||
]; | ||
} | ||
|
||
public function toDatabase(mixed $notifiable): array | ||
{ | ||
return [ | ||
'message' => $this->message, | ||
]; | ||
} | ||
} | ||
``` | ||
|
||
--- | ||
|
||
```php | ||
// Your controller or service | ||
// 通知一条消息 | ||
$user->notify(new TestNotification('系统通知:xxx')); | ||
$noReadCount = $user->unreadNotifications()->count(); | ||
$this->output->success('发送成功,未读消息数:' . $noReadCount); | ||
$notifications = $user->unreadNotifications()->first(); | ||
$this->output->success('消息内容:' . $notifications->data['message']); | ||
$notifications->markAsRead(); | ||
$noReadCount = $user->unreadNotifications()->count(); | ||
$this->output->success('标记已读,未读消息数:' . $noReadCount); | ||
``` | ||
|
||
### SMS Notifications | ||
|
||
- [notification-easy-sms](https://github.com/friendsofhyperf/notification-easysms) | ||
|
||
### Symfony Notifications | ||
|
||
Send notifications using Symfony Notifier. | ||
|
||
Email, SMS, Slack, Telegram, etc. | ||
|
||
```shell | ||
composer require symfony/notifier | ||
``` | ||
|
||
|
||
```shell | ||
composer require symfony/mailer | ||
``` | ||
|
||
--- | ||
|
||
```php | ||
<?php | ||
// app/Factory/Notifier.php | ||
namespace App\Factory; | ||
|
||
use Hyperf\Contract\StdoutLoggerInterface; | ||
use Psr\EventDispatcher\EventDispatcherInterface; | ||
use Symfony\Component\Mailer\Transport; | ||
use Symfony\Component\Notifier\Channel\ChannelInterface; | ||
use Symfony\Component\Notifier\Channel\EmailChannel; | ||
use function Hyperf\Support\env; | ||
|
||
class Notifier | ||
{ | ||
public function __construct( | ||
protected EventDispatcherInterface $dispatcher, | ||
protected StdoutLoggerInterface $logger, | ||
) | ||
{ | ||
} | ||
|
||
public function __invoke() | ||
{ | ||
return new \Symfony\Component\Notifier\Notifier($this->channels()); | ||
} | ||
|
||
/** | ||
* @return ChannelInterface[] | ||
*/ | ||
public function channels(): array | ||
{ | ||
return [ | ||
'email' => new EmailChannel( | ||
transport: Transport::fromDsn( | ||
// MAIL_DSN=smtp://user:password@localhost:1025 | ||
env('MAIL_DSN'), | ||
dispatcher: $this->dispatcher, | ||
logger: $this->logger | ||
), | ||
from: '[email protected]' | ||
), | ||
]; | ||
} | ||
} | ||
``` | ||
|
||
```php | ||
<?php | ||
// app/Notification/TestNotification.php | ||
namespace App\Notification; | ||
|
||
use App\Model\User; | ||
use FriendsOfHyperf\Notification\Notification; | ||
use Symfony\Component\Notifier\Recipient\Recipient; | ||
|
||
class TestNotification extends Notification | ||
{ | ||
public function __construct( | ||
private string $message | ||
){} | ||
|
||
public function via() | ||
{ | ||
return [ | ||
'symfony' | ||
]; | ||
} | ||
|
||
public function toSymfony(User $user) | ||
{ | ||
return (new \Symfony\Component\Notifier\Notification\Notification($this->message,['email']))->content('The introduction to the notification.'); | ||
} | ||
|
||
public function toRecipient(User $user) | ||
{ | ||
return new Recipient('[email protected]'); | ||
} | ||
|
||
|
||
} | ||
``` | ||
|
||
```php | ||
<?php | ||
// config/autoload/dependencies.php | ||
declare(strict_types=1); | ||
/** | ||
* This file is part of Hyperf. | ||
* | ||
* @link https://www.hyperf.io | ||
* @document https://hyperf.wiki | ||
* @contact [email protected] | ||
* @license https://github.com/hyperf/hyperf/blob/master/LICENSE | ||
*/ | ||
return [ | ||
\Symfony\Component\Notifier\NotifierInterface::class => \App\Factory\Notifier::class | ||
]; | ||
|
||
``` | ||
|
||
#### 在控制器中使用 | ||
|
||
```php | ||
$user = User::create(); | ||
// 通知一条消息 | ||
$user->notify(new TestNotification('系统通知:xxx')); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,8 +1,68 @@ | ||
# openai-client | ||
# Hyperf OpenAI Client | ||
|
||
## 安装 | ||
------ | ||
|
||
**OpenAI PHP** for Laravel 是一个功能强大的社区 PHP API 客户端,允许您与 [Open AI API](https://beta.openai.com/docs/api-reference/introduction) 进行交互。 | ||
|
||
> **注意:** 此仓库包含 **OpenAI PHP** 的 Hyperf 集成代码。如果您想在与框架无关的方式中使用 **OpenAI PHP** 客户端,请查看 [openai-php/client](https://github.com/openai-php/client) 仓库。 | ||
## 快速开始 | ||
|
||
> **Requires [PHP 8.1+](https://php.net/releases/)** | ||
首先,通过 [Composer](https://getcomposer.org/) 包管理器安装 OpenAI: | ||
|
||
```shell | ||
composer require friendsofhyperf/openai-client | ||
``` | ||
|
||
接下来,发布配置文件: | ||
|
||
```shell | ||
php bin/hyperf.php vendor:publish friendsofhyperf/openai-client | ||
``` | ||
|
||
这将在您的项目中创建一个 `config/autoload/openai.php` 配置文件,您可以使用环境变量根据需要进行修改: | ||
|
||
```env | ||
OPENAI_API_KEY=sk-... | ||
``` | ||
|
||
最后,您可以使用容器中的 `OpenAI\Client` 实例来访问 OpenAI API: | ||
|
||
```php | ||
use OpenAI\Client; | ||
|
||
$result = di(OpenAI\Client::class)->completions()->create([ | ||
'model' => 'text-davinci-003', | ||
'prompt' => 'PHP is', | ||
]); | ||
|
||
echo $result['choices'][0]['text']; // an open-source, widely-used, server-side scripting language. | ||
``` | ||
|
||
## Azure | ||
|
||
要使用 Azure OpenAI 服务,必须使用工厂手动构建客户端。 | ||
|
||
```php | ||
$client = OpenAI::factory() | ||
->withBaseUri('{your-resource-name}.openai.azure.com/openai/deployments/{deployment-id}') | ||
->withHttpHeader('api-key', '{your-api-key}') | ||
->withQueryParam('api-version', '{version}') | ||
->make(); | ||
``` | ||
|
||
要使用 Azure,您必须部署一个模型,该模型由 {deployment-id} 标识,已集成到 API 调用中。因此,您不必在调用期间提供模型,因为它已包含在 BaseUri 中。 | ||
|
||
因此,一个基本的示例完成调用将是: | ||
|
||
```php | ||
$result = $client->completions()->create([ | ||
'prompt' => 'PHP is' | ||
]); | ||
``` | ||
|
||
## 官方指南 | ||
|
||
有关使用示例,请查看 [openai-php/client](https://github.com/openai-php/client) 仓库。 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,8 +1,37 @@ | ||
# pest-plugin-hyperf | ||
|
||
> 这是一个 [Pest](https://pestphp.com) 插件,使您的 Hyperf 项目的 Pest 能够在基于 Swoole 的协程环境中运行。 | ||
## 安装 | ||
|
||
```shell | ||
composer require friendsofhyperf/pest-plugin-hyperf | ||
composer require friendsofhyperf/pest-plugin-hyperf --dev | ||
``` | ||
|
||
## 使用 | ||
|
||
```shell | ||
php vendor/bin/pest --coroutine | ||
# or | ||
php vendor/bin/pest --prepend test/prepend.php --coroutine | ||
``` | ||
|
||
- 配置 test/prepend.php | ||
|
||
```php | ||
<?php | ||
require_once __DIR__ . '/../vendor/autoload.php'; | ||
|
||
defined('BASE_PATH') or define('BASE_PATH', dirname(__DIR__, 1)); | ||
|
||
(function () { | ||
\Hyperf\Di\ClassLoader::init(); | ||
|
||
\Hyperf\Context\ApplicationContext::setContainer( | ||
new \Hyperf\Di\Container((new \Hyperf\Di\Definition\DefinitionSourceFactory())()) | ||
); | ||
|
||
// $container->get(Hyperf\Contract\ApplicationInterface::class); | ||
})(); | ||
|
||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,8 +1,36 @@ | ||
# pretty-console | ||
# Pretty Console | ||
|
||
The pretty console component for Hyperf. | ||
|
||
[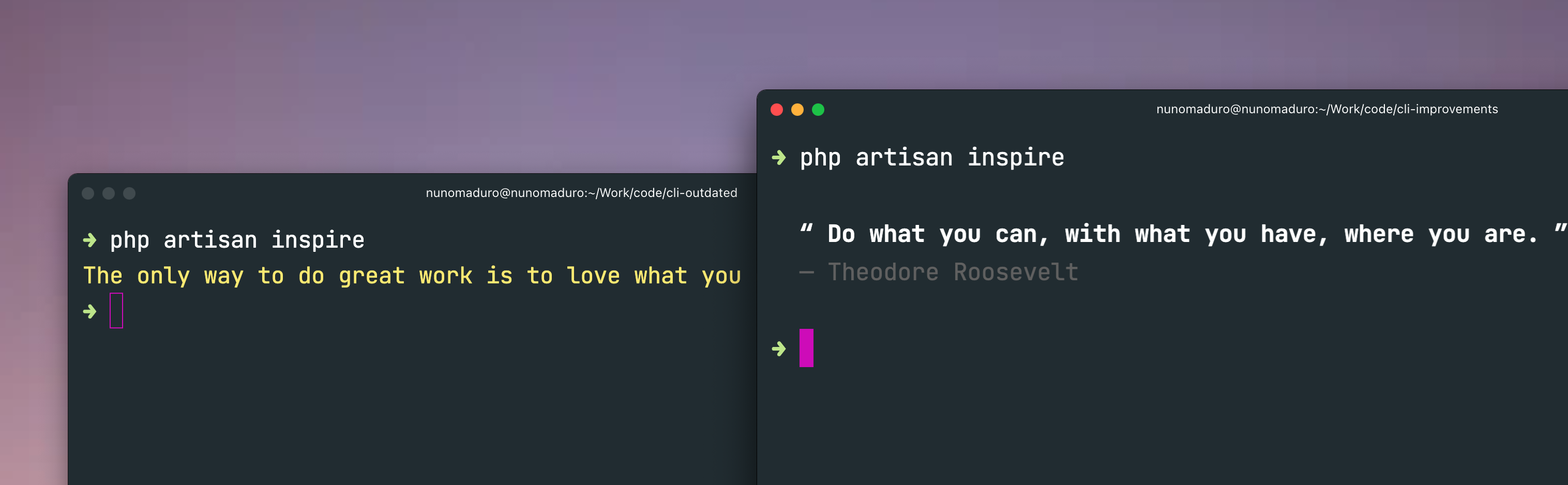](#) | ||
|
||
## 安装 | ||
|
||
```shell | ||
composer require friendsofhyperf/pretty-console | ||
``` | ||
|
||
## 使用 | ||
|
||
```php | ||
<?php | ||
use FriendsOfHyperf\PrettyConsole\Traits\Prettyable; | ||
use Hyperf\Command\Command as HyperfCommand; | ||
use Hyperf\Command\Annotation\Command; | ||
|
||
#[Command] | ||
class FooCommand extends HyperfCommand | ||
{ | ||
use Prettyable; | ||
|
||
public function function handle() | ||
{ | ||
$this->components->info('Your message here.'); | ||
} | ||
} | ||
``` | ||
|
||
## 鸣谢 | ||
|
||
- [nunomaduro/termwind](https://github.com/nunomaduro/termwind) | ||
- [The idea from pr of laravel](https://github.com/laravel/framework/pull/43065) |
Oops, something went wrong.