diff --git a/client-app/src/Components/DonatedItemsList.tsx b/client-app/src/Components/DonatedItemsList.tsx
index c548f0e8..488872ed 100644
--- a/client-app/src/Components/DonatedItemsList.tsx
+++ b/client-app/src/Components/DonatedItemsList.tsx
@@ -226,6 +226,7 @@ const DonatedItemsList: React.FC = () => {
const handleAddNewDonationClick = (): void => {
navigate('/adddonation');
};
+
const downloadBarcode = (id: number) => {
const barcodeElement = document.getElementById(`barcode-${id}`);
if (barcodeElement) {
diff --git a/client-app/src/Components/StatusDisplayPage.js b/client-app/src/Components/StatusDisplayPage.js
deleted file mode 100644
index 155b6ccf..00000000
--- a/client-app/src/Components/StatusDisplayPage.js
+++ /dev/null
@@ -1,174 +0,0 @@
-import React, { useState, useEffect } from 'react';
-import { useLocation, useNavigate } from 'react-router-dom';
-import ItemStatus from '../constants/Enums';
-import '../css/StatusDisplayPage.css';
-
-const StatusDisplayPage = () => {
- const location = useLocation();
- const itemInfo = location.state.itemInfo;
- const navigate = useNavigate();
-
- const [donorInfo, setDonorInfo] = useState({
- fullName: '',
- email: '',
- phone: '',
- address: '',
- status: {
- donated: false,
- inStorageFacility: false,
- refurbished: false,
- received: false,
- sold: false,
- },
- image: null,
- });
-
- // Simulating API call to fetch donor data
- useEffect(() => {
- // Replace with your actual API call logic
- // For now, setting dummy data
- const dummyData = {
- fullName: 'Donor Full Name',
- email: 'donor@example.com',
- phone: '123-456-7890',
- address: '123 Main St',
- status: {
- donated: true,
- inStorageFacility: true,
- refurbished: true,
- received: true,
- sold: true,
- },
- };
- setDonorInfo(dummyData);
- }, []);
-
- const handleCheckboxChange = e => {
- const { name, checked } = e.target;
- setDonorInfo({
- ...donorInfo,
- status: { ...donorInfo.status, [name]: checked },
- });
- };
-
- // Function to handle file upload
- const handleImageUpload = e => {
- const file = e.target.files[0];
- setDonorInfo({
- ...donorInfo,
- image: URL.createObjectURL(file), // Generate a temporary URL for the selected image
- });
- };
- const handleSaveChanges = () => {
- // Logic to handle save changes
- navigate('/Donations'); // Adjust '/donations' to your specific route
- };
-
- const handleCancel = () => {
- navigate('/Donations'); // Adjust '/donations' to your specific route
- };
-
- if (!itemInfo) {
- return
No item information found
;
- }
-
- return (
-
-
-
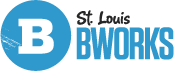
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- {/* Display uploaded image */}
- {donorInfo.image && (
-

- )}
-
-
-
-
-
-
-
-
- );
-};
-
-export default StatusDisplayPage;
diff --git a/client-app/src/css/DonatedItemDetails.css b/client-app/src/css/DonatedItemDetails.css
index a21bb512..eec6c506 100644
--- a/client-app/src/css/DonatedItemDetails.css
+++ b/client-app/src/css/DonatedItemDetails.css
@@ -28,6 +28,16 @@
margin: 0;
}
+.section-header button {
+ margin-left: auto; /* Pushes the button to the right */
+ padding: 5px 10px;
+ background-color: #007bff;
+ color: white;
+ border: none;
+ border-radius: 4px;
+ cursor: pointer;
+}
+
.section-header .icon {
font-size: 28px;
margin-right: 8px;
diff --git a/client-app/src/css/StatusDisplayPage.css b/client-app/src/css/StatusDisplayPage.css
deleted file mode 100644
index 4f692abf..00000000
--- a/client-app/src/css/StatusDisplayPage.css
+++ /dev/null
@@ -1,48 +0,0 @@
-/* BWorks logo styling */
-.bworks-logo {
- display: block;
- margin: 0 auto 20px;
- max-width: 150px;
- /* Adjust the size as needed */
-}
-
-/* Label styling */
-label {
- display: block;
- margin-bottom: 10px;
- font-weight: bold;
-}
-
-/* Input field styling */
-input[type='text'],
-input[type='email'],
-input[type='tel'] {
- width: 100%;
- padding: 10px;
- border: 1px solid #ccc;
- border-radius: 4px;
- margin-bottom: 15px;
-}
-
-/* Radio button styling */
-input[type='radio'] {
- margin-right: 10px;
- vertical-align: middle;
-}
-
-/* Buttons styling */
-button {
- background-color: #0074d9;
- color: #fff;
- border: none;
- padding: 10px 20px;
- border-radius: 4px;
- cursor: pointer;
-}
-
-button[type='submit'] {
- background-color: #2ecc40;
-}
-
-/* Additional styling as needed */
-/* ... */
diff --git a/server/src/schemas/donatedItemSchema.ts b/server/src/schemas/donatedItemSchema.ts
index b3c612e7..81a2f19f 100644
--- a/server/src/schemas/donatedItemSchema.ts
+++ b/server/src/schemas/donatedItemSchema.ts
@@ -1,9 +1,10 @@
import Joi from 'joi';
+
//DonatedItem schema
export const donatedItemSchema = Joi.object({
itemType: Joi.string().required(),
currentStatus: Joi.string()
- .valid('Received', 'Pending', 'Processed', 'Delivered')
+ .valid('Received', 'Donated', 'In storage facility', 'Refurbished', 'Item sold')
.required(),
donorId: Joi.alternatives(Joi.number(), Joi.string().pattern(/^\d+$/))
.required()
@@ -24,7 +25,7 @@ export const donatedItemSchema = Joi.object({
// DonatedItemStatus schema
export const donatedItemStatusSchema = Joi.object({
statusType: Joi.string()
- .valid('Received', 'Pending', 'Processed', 'Delivered')
+ .valid('Received', 'Donated', 'In storage facility', 'Refurbished', 'Item sold')
.required(),
donatedItemId: Joi.number().integer().required(), // Ensures it's a valid integer
dateModified: Joi.date(), // Validates as a proper date